We can make a grouped bar chart with Chart.js by creating a bar chart that has multiple datasets
entries.
To start, we first include the Chart.js library. We also include the moment.js library for formatting dates.
The grouped bar chart will be rendered in a canvas element.
So we write the following code to include all that:
<script src='https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.9.3/Chart.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.24.0/moment.min.js'></script>
<canvas id="barChart" width="400" height="400"></canvas>
Next, we add the code for rendering the grouped bar chart.
We write the following:
const ctx = document.getElementById('barChart').getContext('2d');
const chart = new Chart(ctx, {
type: 'bar',
data: {
labels: [
moment(new Date(2020, 1, 1)).format('YYYY-MM-DD'),
moment(new Date(2020, 1, 2)).format('YYYY-MM-DD'),
moment(new Date(2020, 1, 3)).format('YYYY-MM-DD')
],
datasets: [{
label: '# of Red Votes',
data: [12, 18, 22],
borderWidth: 1,
backgroundColor: ['red', 'red', 'red']
},
{
label: '# of Green Votes',
data: [12, 2, 13],
borderWidth: 1,
backgroundColor: ['green', 'green', 'green']
}
]
},
options: {
scales: {
yAxes: [{
ticks: {
beginAtZero: true
}
}]
}
}
});
The code above has the labels
property with the labels for the x-axis.
The datasets
property has the data sets we display on the y-axis.
data
has the bar heights for each bar.
label
has the label for each bar.
backgroundColor
has the background color for each bar.
borderWidth
has the border width for each bar.
Then in the options
property, we have the beginAtZero
property to make sure that the y-axis starts at zero instead of the value of the lowest bar value.
In the end, we have:
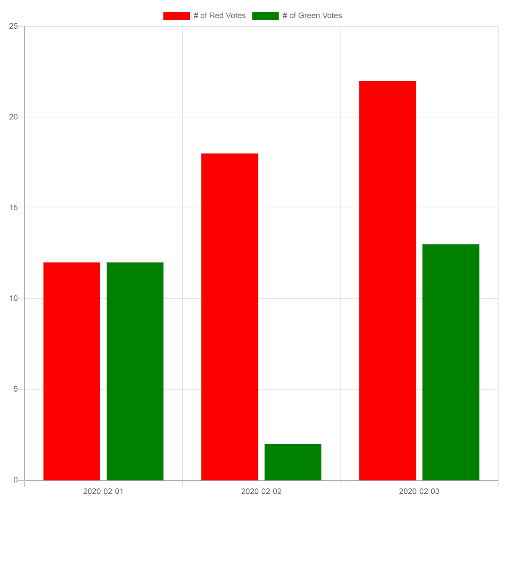
It’s a grouped bar chart with red and green bars displaying the data in the data
arrays.
With Chart.js, creating a grouped bar chart is just a matter of setting the labels, bar heights, and bar colors of each bar.