react-chartjs-2 is an easy to use library for creating all kinds of charts. It’s based on Chart.js, which renders chart in an HTML canvas element.
We can use it to render charts in a canvas inside a React app. To get started, we install Chart.js and react-chartjs-2 by running:
npm install --save react-chartjs-2 chart.js moment
We also installed moments to create dates for the x-axis labels. Then we can write the following code:
import React from "react";
import { Line } from "react-chartjs-2";
import moment from "moment";
const startDate = new Date(2020, 0, 1);
const labels = [];
for (let i = 0; i < 6; i++) {
const date = moment(startDate)
.add(i, "days")
.format("YYYY-MM-DD");
labels.push(date.toString());
}
const data = canvas => {
const ctx = canvas.getContext("2d");
const gradient = ctx.createLinearGradient(0, 0, 100, 0);
return {
backgroundColor: gradient,
labels,
datasets: [
{
label: "# of Votes",
data: [12, 19, 3, 5, 2, 3],
borderWidth: 3,
fill: false,
borderColor: "green"
}
]
};
};
export default function App() {
return (
<div className="App">
<Line data={data} />
</div>
);
}
We first create the x-axis labels and populate them in the labels
array/
We did that by using the moment
function and call add
on it to add 1 day in each iteration of the for
loop.
Then we create a data
function, which takes the canvas
object, which has the canvas element as the parameter.
Then we get the canvas from it and change the items that we want.
The function returns the options for our graph, including the data.
The object we return in data
has various options.
It has the labels
property to populate the x-axis labels.
backgroundColor
has the color for our graph.
datasets
has an array with an object with the data and some options for the line.
data
has the y-axis values for each x-axis value.
borderWidth
specifies the thickness of the line in pixels.
fill
is set to false
means that we don’t have any colors between the x-axis and our line.
label
is the text label for our legend.
borderColor
is the color of our line.
Once we have that code, we’ll see the following graph:
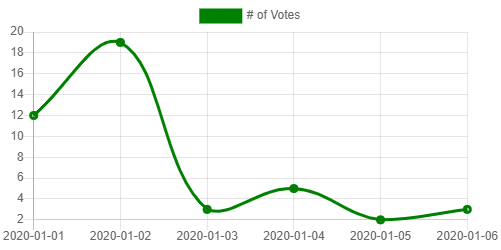