We can create stacked bar chart with Chart.js
With the stacked
option in Chart.js, we can create a stacked bar chart from a simple bar chart.
To create the chart, first we need to add Chart.js to our code by writing:
<script src='https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.9.3/Chart.min.js'></script>
<script src='https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.24.0/moment.min.js'></script>
<canvas id="barChart" width="400" height="400"></canvas>
The code above also adds moment.js for manipulating time and a canvas element to display the chart.
Then we write:
const ctx = document.getElementById('barChart').getContext('2d');
const chart = new Chart(ctx, {
type: 'bar',
data: {
labels: [
moment(new Date(2020, 0, 1)).format('YYYY-MM-DD'),
moment(new Date(2020, 0, 2)).format('YYYY-MM-DD'),
moment(new Date(2020, 0, 3)).format('YYYY-MM-DD')
],
datasets: [{
label: '# of Green Votes',
data: [12, 19, 13],
borderWidth: 1,
backgroundColor: ['green', 'green', 'green']
},
{
label: '# of Pink Votes',
data: [15, 9, 19],
borderWidth: 1,
backgroundColor: ['pink', 'pink', 'pink']
}
]
},
options: {
scales: {
xAxes: [{
stacked: true
}],
yAxes: [{
stacked: true
}]
}
}
});
We’ve specified by the labels in the labels
property to be 3 dates.
In the datasets
property, we have the label
to display the label of the legtnd.
The borderWidth
has the border with value of the bar in pixels.
The backgroundColor
has the background color of the bars.
The data
has the bar heights of the bars.
The options
object has the property that lets us create a stacked bar chart.
We have the xAxes
property, which has an array with an object that has stacked
set to true
.
Also, we have the same property in the object in the yAxes
array.
Once we have that, we get:
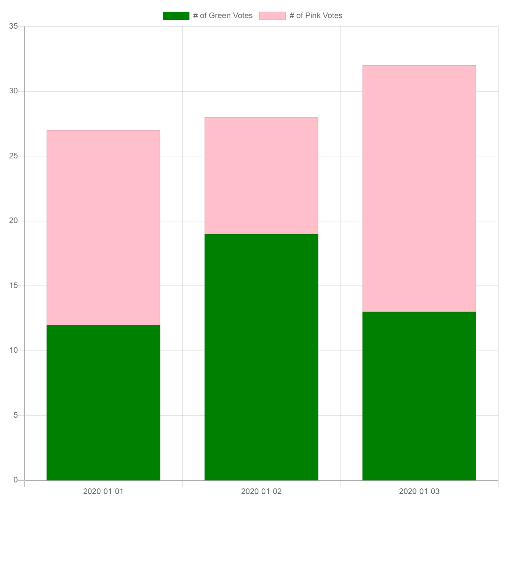
Creating a stacked bar chart is just as easy as add the data to display for each bar.
Then we set the stacked
option in the options
object to make 2 or more bars stacked together.