Here, we will see how to check internet connection status when we are working online or offline using javascript with bootstrap stylesheet. In web development display of internet connection status is very useful instead of using push notification. It is very effective, when we are surfing the website in the middle of an internet connection lost at that time this toast notification indicates the internet connection status. Here, we will use a lightweight javascript toast component and bootstrap 4 library to display the status.
Follow the codes below,
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Toast Notification for Internet Connection Check Using Bootstrap 4 & javascript</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap-icons@1.4.1/font/bootstrap-icons.css">
</head>
<body>
<div class="container">
<br />
<br />
<h1 align="center">Toast Notification for Internet Connection Check Using Bootstrap 4 & javascript</h1>
<br />
<h4>Our other blogs</h4>
<table class="table-border">
<thead>
<tr>
<th>Post Title</th>
<th class="p-2">View Post</th>
</tr>
</thead>
<tbody>
<tr>
<td>
JavaScript Multiple File Upload Progress Bar Using Ajax With PHP
</td>
<td class="p-2"><a href="https://codeamend.com/blog/javascript-multiple-file-upload-progress-bar-using-ajax-with-php/">View Blog</a></td>
</tr>
<tr>
<td>
ERROR in ngcc is already running at process with id 6139
</td>
<td class="p-2"><a href="https://codeamend.com/blog/error-in-ngcc-is-already-running-at-process-with-id-6139/">View Blog</a></td>
</tr>
<tr>
<td>
Create Google Client ID & Secret from Google developer console
</td>
<td class="p-2"><a href="https://codeamend.com/blog/create-google-client-id-secret-from-google-developer-console/">View Blog</a></td>
</tr>
<tr>
<td>
Add Content Below the “Place Order” Button in WooCommerce checkout page
</td>
<td class="p-2"><a href="https://codeamend.com/blog/add-content-below-the-place-order-button-in-woocommerce-checkout-page/">View Blog</a></td>
</tr>
<tr>
<td>
Upload Image From URL in PHP
</td>
<td class="p-2"><a href="https://codeamend.com/blog/upload-image-from-url-in-php/">View Blog</a></td>
</tr>
<tr>
<td>
jQuery Chaining Method
</td>
<td class="p-2"><a href="https://codeamend.com/blog/jquery-chaining-method/">View Blog</a></td>
</tr>
<tr>
<td>
Google Login or Sign In with Angular Application
</td>
<td class="p-2"><a href="https://codeamend.com/blog/google-login-or-sign-in-with-angular-application/">View Blog</a></td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
<div class="toast" style="position: absolute; top: 25px; right: 25px;">
<div class="toast-header">
<i class="bi bi-wifi"></i>
<strong class="mr-auto"><span class="text-success">You're online now</span></strong>
<button type="button" class="ml-2 mb-1 close" data-dismiss="toast" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="toast-body">
Your internet is connected.
</div>
</div>
<script>
var network_status = 'online';
var current_network_status = 'online';
function check_internet_connection(){
if(navigator.onLine){
network_status = 'online';
}
else
{
network_status = 'offline';
}
if(network_status == 'online')
{
$('i.bi').addClass('bi-wifi');
$('i.bi').removeClass('bi-wifi-off');
$('.mr-auto').html("<span class='text-success'>You are online now</span>");
$('.toast-body').text('Your internet is connected.');
}
else
{
$('i.bi').addClass('bi-wifi-off');
$('i.bi').removeClass('bi-wifi');
$('.mr-auto').html("<span class='text-danger'>You are offline now</span>");
$('.toast-body').text('Opps! your internet is disconnected.')
}
current_network_status = network_status;
$('.toast').toast({
autohide:false
});
$('.toast').toast('show');
}
check_internet_connection();
setInterval(function(){
check_internet_connection();
}, 1000);
</script>
Output
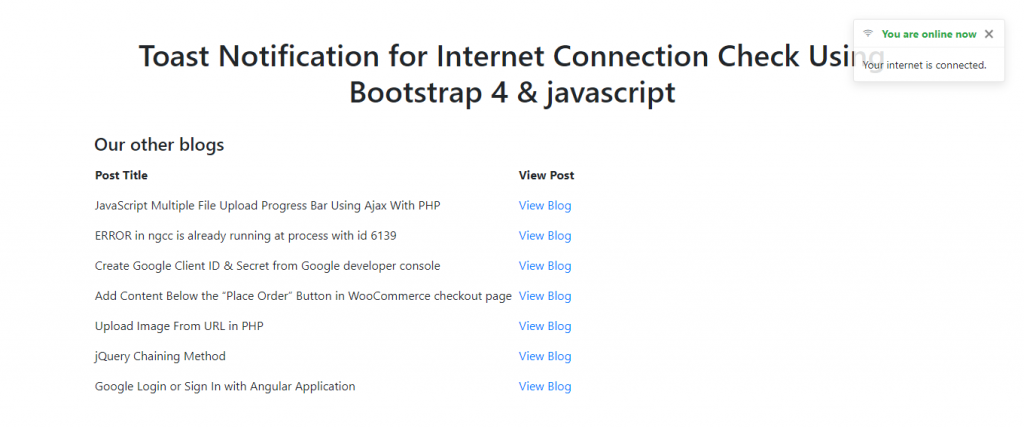
Total Views: 1,735